Python Templates
This template engines site contains a range of information from what templates engines are to listing more esoteric Python template engines. Python Template Engine Comparison is an older but still relevant post by the creator of Jinja that explains why and how he switches between Mako, Jinja and Genshi for various projects he works on. Contents; Using Jinja2 Templates. This part of the Python Guestbook code walkthrough shows how to use Jinja templates to generate dynamic web content. This page is part of a multi-page tutorial. To start from the beginning and see instructions for setting up, go to Creating a Guestbook. HTML embedded in code is messy.
Time to display some data! Django gives us some helpful built-in template tags for that.
What are template tags?
You see, in HTML, you can't really write Python code, because browsers don't understand it. They know only HTML. We know that HTML is rather static, while Python is much more dynamic.
Django template tags allow us to transfer Python-like things into HTML, so you can build dynamic websites faster. Cool!
Display post list template
In the previous chapter we gave our template a list of posts in the posts
variable. Now we will display it in HTML.
To print a variable in Django templates, we use double curly brackets with the variable's name inside, like this:
blog/templates/blog/post_list.html
Try this in your blog/templates/blog/post_list.html
template. Open it up in the code editor, and replace everything from the second <div>
to the third </div>
with {{ posts }}
. Save the file, and refresh the page to see the results:
As you can see, all we've got is this:
blog/templates/blog/post_list.html
This means that Django understands it as a list of objects. Remember from Introduction to Python how we can display lists? Yes, with for loops! In a Django template you do them like this:
blog/templates/blog/post_list.html
Try this in your template.
It works! But we want the posts to be displayed like the static posts we created earlier in the Introduction to HTML chapter. You can mix HTML and template tags. Our body
will look like this:
blog/templates/blog/post_list.html
Everything you put between {% for %}
and {% endfor %}
will be repeated for each object in the list. Refresh your page:
Have you noticed that we used a slightly different notation this time ({{ post.title }}
or {{ post.text }}
)? We are accessing data in each of the fields defined in our Post
model. Also, the linebreaksbr
is piping the posts' text through a filter to convert line-breaks into paragraphs.
One more thing
It'd be good to see if your website will still be working on the public Internet, right? Let's try deploying to PythonAnywhere again. Here's a recap of the steps…
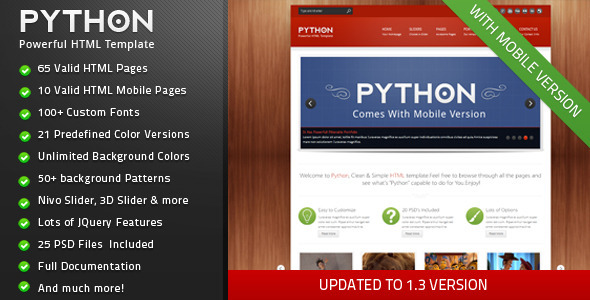
- First, push your code to GitHub
command-line
- Then, log back in to PythonAnywhere and go to your Bash console (or start a new one), and run:
PythonAnywhere command-line
(Remember to substitute <your-pythonanywhere-domain>
with your actual PythonAnywhere subdomain, without the angle-brackets.)
- Finally, hop on over to the 'Web' page and hit Reload on your web app. (To reach other PythonAnywhere pages from the console, use the menu button in the upper right corner.) Your update should be live on https://subdomain.pythonanywhere.com -- check it out in the browser! If the blog posts on your PythonAnywhere site don't match the posts appearing on the blog hosted on your local server, that's OK. The databases on your local computer and Python Anywhere don't sync with the rest of your files.
Congrats! Now go ahead and try adding a new post in your Django admin (remember to add published_date!) Make sure you are in the Django admin for your pythonanywhere site, https://subdomain.pythonanywhere.com/admin. Then refresh your page to see if the post appears there.
Works like a charm? We're proud! Step away from your computer for a bit – you have earned a break. :)
- Flask Tutorial
- Flask Useful Resources
- Selected Reading
It is possible to return the output of a function bound to a certain URL in the form of HTML. For instance, in the following script, hello() function will render ‘Hello World’ with <h1> tag attached to it.
However, generating HTML content from Python code is cumbersome, especially when variable data and Python language elements like conditionals or loops need to be put. This would require frequent escaping from HTML.
This is where one can take advantage of Jinja2Wintv v7 serial number. template engine, on which Flask is based. Instead of returning hardcode HTML from the function, a HTML file can be rendered by the render_template() function.
Flask will try to find the HTML file in the templates folder, in the same folder in which this script is present.
- Application folder
- Hello.py
- templates
- hello.html
The term ‘web templating system’ refers to designing an HTML script in which the variable data can be inserted dynamically. A web template system comprises of a template engine, some kind of data source and a template processor.
Flask uses jinga2 template engine. A web template contains HTML syntax interspersed placeholders for variables and expressions (in these case Python expressions) which are replaced values when the template is rendered.
The following code is saved as hello.html in the templates folder.
Next, run the following script from Python shell.
As the development server starts running, open the browser and enter URL as − http://localhost:5000/hello/mvl
The variable part of URL is inserted at {{ name }} place holder.
The Jinga2 template engine uses the following delimiters for escaping from HTML.
- {% .. %} for Statements
- {{ .. }} for Expressions to print to the template output
- {# .. #} for Comments not included in the template output
- # .. ## for Line Statements
In the following example, use of conditional statement in the template is demonstrated. The URL rule to the hello() function accepts the integer parameter. It is passed to the hello.html template. Inside it, the value of number received (marks) is compared (greater or less than 50) and accordingly HTML is conditionally rendered.
Python Templates String
The Python Script is as follows −
HTML template script of hello.html is as follows −
Note that the conditional statements if-else and endif are enclosed in delimiter {%.%}.
Run the Python script and visit URL http://localhost/hello/60 and then http://localhost/hello/30 to see the output of HTML changing conditionally.
The Python loop constructs can also be employed inside the template. In the following script, the result() function sends a dictionary object to template results.html when URL http://localhost:5000/result is opened in the browser.
The Template part of result.html employs a for loop to render key and value pairs of dictionary object result{} as cells of an HTML table.
Run the following code from Python shell.
Save the following HTML script as result.html in the templates folder.
Here, again the Python statements corresponding to the For loop are enclosed in {%.%} whereas, the expressions key and value are put inside {{ }}.
Free Python Templates
After the development starts running, open http://localhost:5000/result in the browser to get the following output.