Python Pyserial Readline Example
Dec 15, 2017 I am using python 2.7.2 with pyserial 2.6. What is the best way to use pyserial.readline when talking to a device that has a character other than 'n' for eol? The pyserial doc points out that py.
All your code in one place
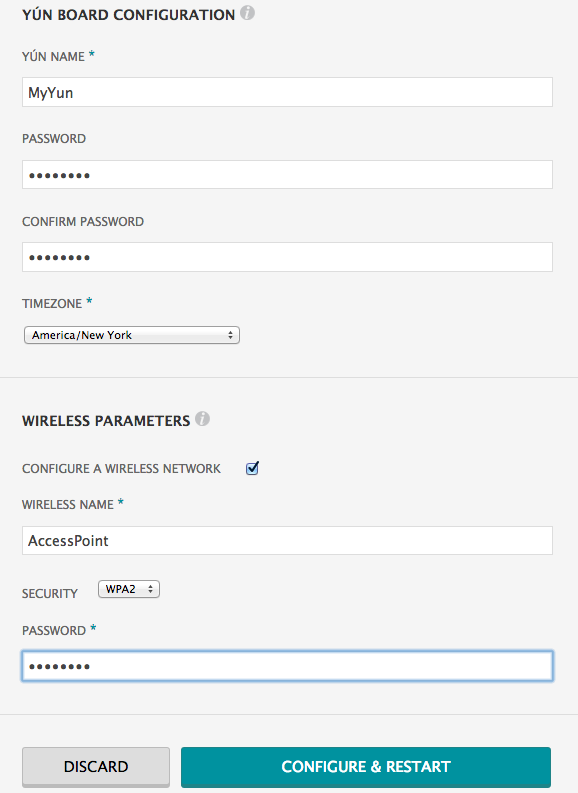
GitHub makes it easy to scale back on context switching. Read rendered documentation, see the history of any file, and collaborate with contributors on projects across GitHub.
Sign up for free See pricing for teams and enterprises1 contributor
#! /usr/bin/env python |
# |
# This file is part of pySerial - Cross platform serial port support for Python |
# (C) 2010-2015 Chris Liechti <cliechti@gmx.net> |
# |
# SPDX-License-Identifier: BSD-3-Clause |
'' |
Some tests for the serial module. |
Part of pyserial (http://pyserial.sf.net) (C)2010 cliechti@gmx.net |
Intended to be run on different platforms, to ensure portability of |
the code. |
For all these tests a simple hardware is required. |
Loopback HW adapter: |
Shortcut these pin pairs: |
TX <-> RX |
RTS <-> CTS |
DTR <-> DSR |
On a 9 pole DSUB these are the pins (2-3) (4-6) (7-8) |
'' |
import unittest |
import sys |
import serial |
#~ print serial.VERSION |
# on which port should the tests be performed: |
PORT='loop://' |
if sys.version_info >= (3, 0): |
defdata(string): |
returnbytes(string, 'latin1') |
else: |
defdata(string): |
return string |
classTest_Readline(unittest.TestCase): |
''Test readline function'' |
defsetUp(self): |
self.s = serial.serial_for_url(PORT, timeout=1) |
deftearDown(self): |
self.s.close() |
deftest_readline(self): |
''Test readline method'' |
self.s.write(serial.to_bytes([0x31, 0x0a, 0x32, 0x0a, 0x33, 0x0a])) |
self.assertEqual(self.s.readline(), serial.to_bytes([0x31, 0x0a])) |
self.assertEqual(self.s.readline(), serial.to_bytes([0x32, 0x0a])) |
self.assertEqual(self.s.readline(), serial.to_bytes([0x33, 0x0a])) |
# this time we will get a timeout |
self.assertEqual(self.s.readline(), serial.to_bytes([])) |
deftest_readlines(self): |
''Test readlines method'' |
self.s.write(serial.to_bytes([0x31, 0x0a, 0x32, 0x0a, 0x33, 0x0a])) |
self.assertEqual( |
self.s.readlines(), |
[serial.to_bytes([0x31, 0x0a]), serial.to_bytes([0x32, 0x0a]), serial.to_bytes([0x33, 0x0a])] |
) |
deftest_xreadlines(self): |
''Test xreadlines method (skipped for io based systems)'' |
ifhasattr(self.s, 'xreadlines'): |
self.s.write(serial.to_bytes([0x31, 0x0a, 0x32, 0x0a, 0x33, 0x0a])) |
self.assertEqual( |
list(self.s.xreadlines()), |
[serial.to_bytes([0x31, 0x0a]), serial.to_bytes([0x32, 0x0a]), serial.to_bytes([0x33, 0x0a])] |
) |
deftest_for_in(self): |
''Test for line in s'' |
self.s.write(serial.to_bytes([0x31, 0x0a, 0x32, 0x0a, 0x33, 0x0a])) |
lines = [] |
for line inself.s: |
lines.append(line) |
self.assertEqual( |
lines, |
[serial.to_bytes([0x31, 0x0a]), serial.to_bytes([0x32, 0x0a]), serial.to_bytes([0x33, 0x0a])] |
) |
deftest_alternate_eol(self): |
''Test readline with alternative eol settings (skipped for io based systems)'' |
ifhasattr(self.s, 'xreadlines'): # test if it is our FileLike base class |
self.s.write(serial.to_bytes('nornonyesrn')) |
self.assertEqual( |
self.s.readline(eol=serial.to_bytes('rn')), |
serial.to_bytes('nornonyesrn')) |
if__name__'__main__': |
import sys |
sys.stdout.write(__doc__) |
iflen(sys.argv) >1: |
PORT= sys.argv[1] |
sys.stdout.write('Testing port: {!r}n'.format(PORT)) |
sys.argv[1:] = ['-v'] |
# When this module is executed from the command-line, it runs all its tests |
unittest.main() |
Copy lines Copy permalink
Miniterm
Miniterm is now available as module instead of example.see :ref:`miniterm` for details.
- miniterm.py
- The miniterm program.
- setup-miniterm-py2exe.py
- This is a py2exe setup script for Windows. It can be used to create astandalone
miniterm.exe
.
TCP/IP - serial bridge
This program opens a TCP/IP port. When a connection is made to that port (e.g.with telnet) it forwards all data to the serial port and vice versa.
This example only exports a raw socket connection. The next examplebelow gives the client much more control over the remote serial port.
- The serial port settings are set on the command line when starting theprogram.
- There is no possibility to change settings from remote.
- All data is passed through as-is.
- tcp_serial_redirect.py
- Main program.
Single-port TCP/IP - serial bridge (RFC 2217)
Simple cross platform RFC 2217 serial port server. It uses threads and isportable (runs on POSIX, Windows, etc).

- The port settings and control lines (RTS/DTR) can be changed at any timeusing RFC 2217 requests. The status lines (DSR/CTS/RI/CD) are polled everysecond and notifications are sent to the client.
- Telnet character IAC (0xff) needs to be doubled in data stream. IAC followedby another value is interpreted as Telnet command sequence.
- Telnet negotiation commands are sent when connecting to the server.
- RTS/DTR are activated on client connect and deactivated on disconnect.
- Default port settings are set again when client disconnects.
- rfc2217_server.py
- Main program.
- setup-rfc2217_server-py2exe.py
- This is a py2exe setup script for Windows. It can be used to create astandalone
rfc2217_server.exe
.
Multi-port TCP/IP - serial bridge (RFC 2217)
This example implements a TCP/IP to serial port service that works withmultiple ports at once. It uses select, no threads, for the serial ports andthe network sockets and therefore runs on POSIX systems only.
- Full control over the serial port with RFC 2217.
- Check existence of
/tty/USB0...8
. This is done every 5 seconds usingos.path.exists
. - Send zeroconf announcements when port appears or disappears (usespython-avahi and dbus). Service name:
_serial_port._tcp
. - Each serial port becomes available as one TCP/IP server. e.g.
/dev/ttyUSB0
is reachable at<host>:7000
. - Single process for all ports and sockets (not per port).
- The script can be started as daemon.
- Logging to stdout or when run as daemon to syslog.
- Default port settings are set again when client disconnects.
- modem status lines (CTS/DSR/RI/CD) are not polled periodically and the servertherefore does not send NOTIFY_MODEMSTATE on its own. However it responds torequest from the client (i.e. use the
poll_modem
option in the URL whenusing a pySerial client.)
Requirements:
- Python (>= 2.4)
- python-avahi
- python-dbus
- python-serial (>= 2.5)
Installation as daemon:
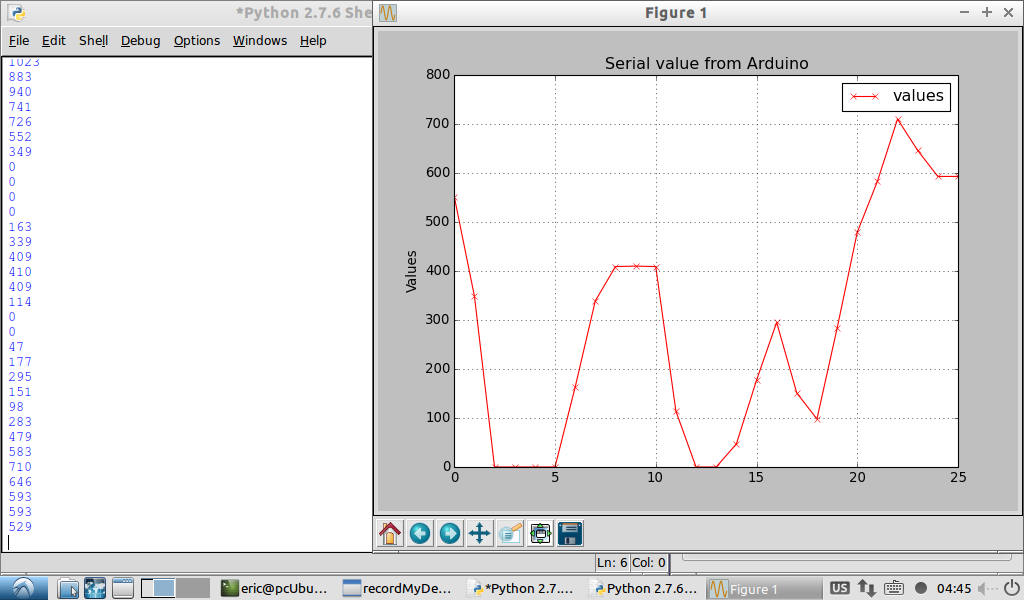
- Copy the script
port_publisher.py
to/usr/local/bin
. - Copy the script
port_publisher.sh
to/etc/init.d
. - Add links to the runlevels using
update-rc.d port_publisher.sh defaults 99
- That's it :-) the service will be started on next reboot. Alternatively run
invoke-rc.d port_publisher.sh start
as root.
- port_publisher.py
- Multi-port TCP/IP-serial converter (RFC 2217) for POSIX environments.
- port_publisher.sh
- Example init.d script.
wxPython examples
Python Pyserial Readline Example Pdf
A simple terminal application for wxPython and a flexible serial portconfiguration dialog are shown here.
Python Pyserial Readline Example Java
- wxTerminal.py
- A simple terminal application. Note that the length of the buffer islimited by wx and it may suddenly stop displaying new input.
- wxTerminal.wxg
- A wxGlade design file for the terminal application.
- wxSerialConfigDialog.py
- A flexible serial port configuration dialog.
- wxSerialConfigDialog.wxg
- The wxGlade design file for the configuration dialog.
- setup-wxTerminal-py2exe.py
- A py2exe setup script to package the terminal application.
Unit tests
The project uses a number of unit test to verify the functionality. They allneed a loop back connector. The scripts itself contain more information. Alltest scripts are contained in the directory test
.
The unit tests are performed on port loop://
unless a different devicename or URL is given on the command line (sys.argv[1]
). e.g. to run thetest on an attached USB-serial converter hwgrep://USB
could be used orthe actual name such as /dev/ttyUSB0
or COM1
(depending on platform).
- run_all_tests.py
- Collect all tests from all
test*
files and run them. By default, theloop://
device is used. - test.py
- Basic tests (binary capabilities, timeout, control lines).
- test_advanced.py
- Test more advanced features (properties).
- test_high_load.py
- Tests involving sending a lot of data.
- test_readline.py
- Tests involving
readline
. - test_iolib.py
- Tests involving the :mod:`io` library. Only available for Python 2.6 andnewer.
- test_url.py
- Tests involving the :ref:`URL <URLs>` feature.